Your cart is currently empty!
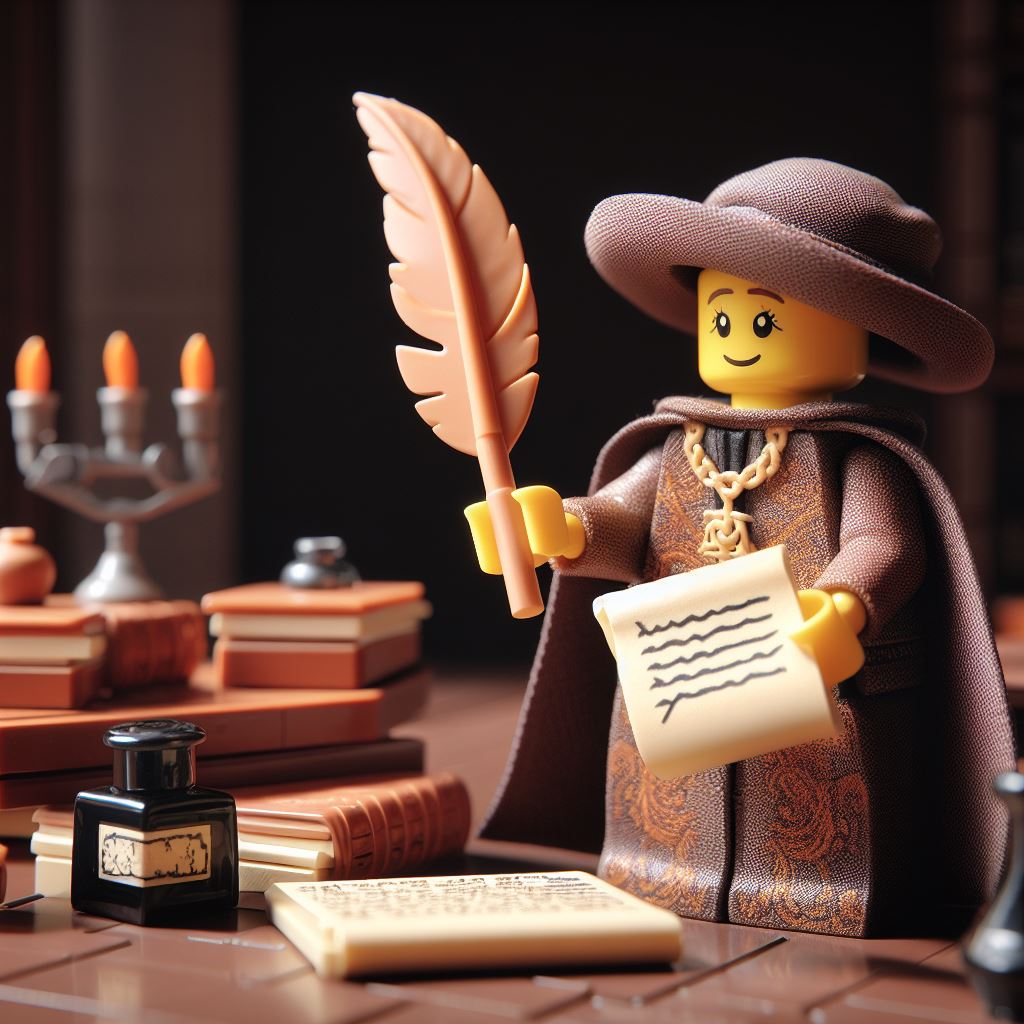
String Literal Cheat Sheet (Python, C#, JS)
Python
Python uses f-strings for string interpolation. Here’s an example:
name = "Alice"
age = 25
print(f"Hello, my name is {name} and I'm {age} years old.")
In this code, the variables name
and age
are inserted into the string using curly braces {}
.
C#
C# uses string interpolation with the $
symbol. Here’s an example:
string name = "Alice";
int age = 25;
Console.WriteLine($"Hello, my name is {name} and I'm {age} years old.");
In this code, the variables name
and age
are inserted into the string using curly braces {}
.
JavaScript
JavaScript uses template literals for string interpolation. Template literals are enclosed by the backtick (
) character instead of double or single quotes. Here’s an example:
let name = "Alice";
let age = 25;
console.log(`Hello, my name is ${name} and I'm ${age} years old.`);
In this code, the variables name
and age
are inserted into the string using ${}
.
I hope this helps! Drop a comment if you would like some more cheat sheets or other suggestions!
by
Tags:
Leave a Reply